Ground Models
The ground models in PyWake are used to model the effects that the ground has on the inflow and wake.
Install PyWake if needed
[1]:
# Install PyWake if needed
try:
import py_wake
except ModuleNotFoundError:
!pip install git+https://gitlab.windenergy.dtu.dk/TOPFARM/PyWake.git
Mirror
The Mirror ground model lets the ground mirror the wake deficit. It is implemented by adding wakes from underground mirrored wind turbines
[2]:
import numpy as np
import matplotlib.pyplot as plt
[3]:
from py_wake.ground_models import Mirror
from py_wake import NOJ
from py_wake.flow_map import YZGrid
from py_wake.examples.data.hornsrev1 import V80, Hornsrev1Site
site = Hornsrev1Site()
windTurbines = V80()
wt_x, wt_y = site.initial_position.T
wfm = NOJ(site, windTurbines, k=.5, groundModel=Mirror())
plt.figure()
wfm([0], [0], wd=0).flow_map(YZGrid(x=0, y=np.arange(-300, 100, 1) + .1, z=np.arange(-100, 200))).plot_wake_map()
plt.xlabel('x [m]')
plt.ylabel('y [m]')
/builds/TOPFARM/PyWake/py_wake/deficit_models/noj.py:88: UserWarning: The NOJ model is not representative of the setup used in the literature. For this, use py_wake.literature.noj.Jensen_1983 instead
DeprecatedModel.__init__(self, 'py_wake.literature.noj.Jensen_1983')
[3]:
Text(0, 0.5, 'y [m]')
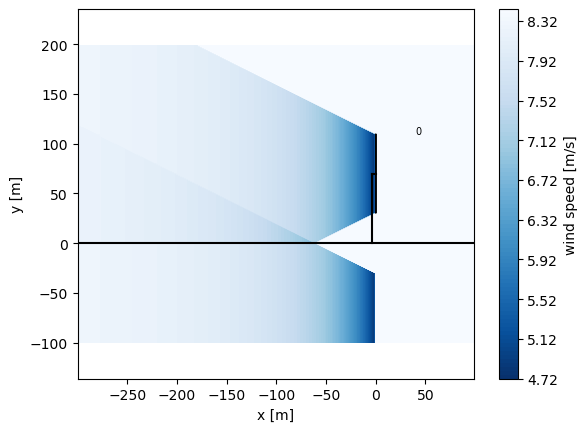